Machineboy空
[From Nand to Tetris] Project 2 - HalfAdder, FullAdder, Add16, Inc16, ALU 본문
Computer/CS
[From Nand to Tetris] Project 2 - HalfAdder, FullAdder, Add16, Inc16, ALU
안녕도라 2025. 1. 23. 14:06만들 것
- HalfAdder
- FullAdder
- Add16
- Inc16
- ALU
기본 개념, 가산기(Adder)란?
컴퓨터의 기본 요소.
논리 대수에 따라서 동작하도록 반도체로 만든 논리 소자를 사용하여 구성한 회로.
입력값에 의해 불대수(boolean algebra)의 값이 출력되는 논리회로.
기억 능력은 가지지 않는다.
반가산기(Half Adder) | 전가산기(Full Adder) |
2진수로 나타낸 수들을 1비트씩 합하여 그 결과로 1비트의 합과 1비트의 자리올림(carry)을 발생하는 회로 | 자릿수가 많은 2진수의 덧셈에서 어떤 자리의 덧셈을 할 때, 낮은 자리로부터의 올림수를 고려한 2진 1자리의 가산기. |
일정한 수의 비트로 나타낸 수의 가산은 불가능 자리올림은 신호로 출력 |
이상은 지식백과 정의인데 무슨 말인지 잘 이해가 가지 않아서 강의를 찾아봤다
https://www.youtube.com/watch?v=F3vyljV4soo
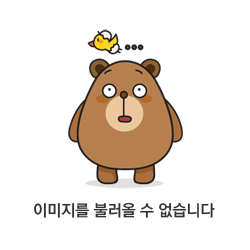
완벽은 아니지만, Project 1때는 논리 연산도 제대로 이해하지 못하고 마구잡이로 했었구나 깨달았다.
HalfAdder
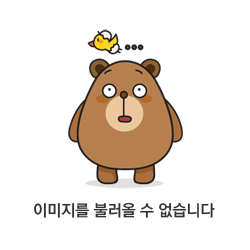
/**
* Computes the sum of two bits.
*/
CHIP HalfAdder {
IN a, b; // 1-bit inputs
OUT sum, // Right bit of a + b
carry; // Left bit of a + b
PARTS:
Xor(a = a, b = b, out = sum);
And(a= a, b= b, out= carry);
}
FullAdder
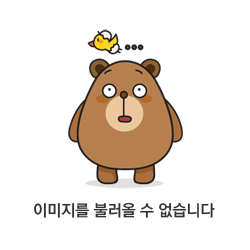
/**
* Computes the sum of three bits.
*/
CHIP FullAdder {
IN a, b, c; // 1-bit inputs
OUT sum, // Right bit of a + b + c
carry; // Left bit of a + b + c
PARTS:
Xor(a = a, b = b, out = temp1);
And(a= a, b= b, out= temp2);
Xor(a = temp1, b = c, out = sum);
And(a= temp1, b= c, out= temp3);
Or(a= temp3, b= temp2, out= carry);
}
Add16
/**
* 16-bit adder: Adds two 16-bit two's complement values.
* The most significant carry bit is ignored.
*/
CHIP Add16 {
IN a[16], b[16];
OUT out[16];
PARTS:
HalfAdder(a=a[0],b=b[0],sum=out[0],carry=c);
FullAdder(a=a[1],b=b[1],c=c,sum=out[1],carry=d);
FullAdder(a=a[2],b=b[2],c=d,sum=out[2],carry=e);
FullAdder(a=a[3],b=b[3],c=e,sum=out[3],carry=f);
FullAdder(a=a[4],b=b[4],c=f,sum=out[4],carry=g);
FullAdder(a=a[5],b=b[5],c=g,sum=out[5],carry=h);
FullAdder(a=a[6],b=b[6],c=h,sum=out[6],carry=i);
FullAdder(a=a[7],b=b[7],c=i,sum=out[7],carry=j);
FullAdder(a=a[8],b=b[8],c=j,sum=out[8],carry=k);
FullAdder(a=a[9],b=b[9],c=k,sum=out[9],carry=l);
FullAdder(a=a[10],b=b[10],c=l,sum=out[10],carry=m);
FullAdder(a=a[11],b=b[11],c=m,sum=out[11],carry=n);
FullAdder(a=a[12],b=b[12],c=n,sum=out[12],carry=o);
FullAdder(a=a[13],b=b[13],c=o,sum=out[13],carry=p);
FullAdder(a=a[14],b=b[14],c=p,sum=out[14],carry=q);
FullAdder(a=a[15],b=b[15],c=q,sum=out[15],carry=drop);
}
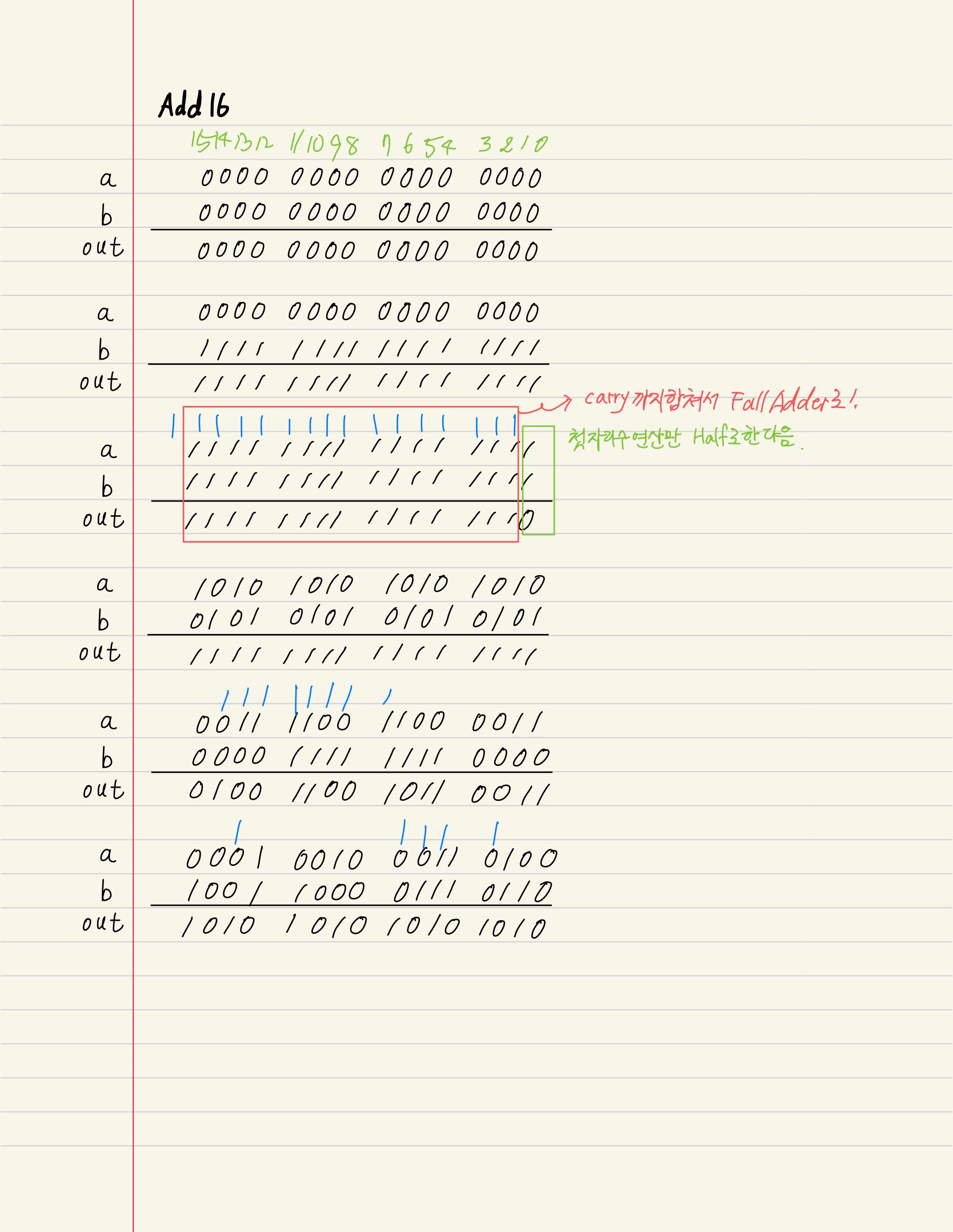
Inc16

/**
* 16-bit incrementer:
* out = in + 1
*/
CHIP Inc16 {
IN in[16];
OUT out[16];
PARTS:
HalfAdder(a=in[0],b= true,sum=out[0],carry=c);
HalfAdder(a=in[1],b= c,sum=out[1],carry=d);
HalfAdder(a=in[2],b= d,sum=out[2],carry=e);
HalfAdder(a=in[3],b= e,sum=out[3],carry=f);
HalfAdder(a=in[4],b= f,sum=out[4],carry=g);
HalfAdder(a=in[5],b= g,sum=out[5],carry=h);
HalfAdder(a=in[6],b= h,sum=out[6],carry=i);
HalfAdder(a=in[7],b= i,sum=out[7],carry=j);
HalfAdder(a=in[8],b= j,sum=out[8],carry=k);
HalfAdder(a=in[9],b= k,sum=out[9],carry=l);
HalfAdder(a=in[10],b= l,sum=out[10],carry=m);
HalfAdder(a=in[11],b= m,sum=out[11],carry=n);
HalfAdder(a=in[12],b= n,sum=out[12],carry=o);
HalfAdder(a=in[13],b= o,sum=out[13],carry=p);
HalfAdder(a=in[14],b= p,sum=out[14],carry=q);
HalfAdder(a=in[15],b= q,sum=out[15],carry=drop);
}
ALU
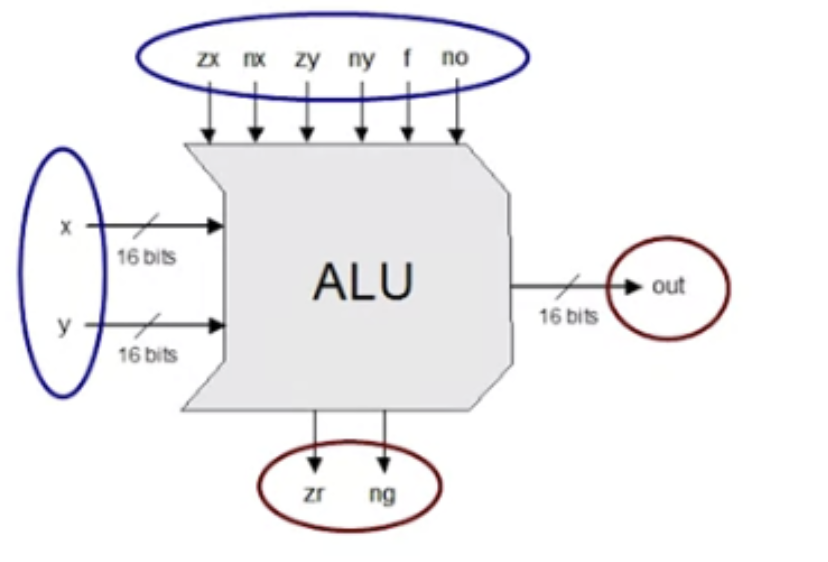
/**
* ALU (Arithmetic Logic Unit):
* Computes out = one of the following functions:
* 0, 1, -1,
* x, y, !x, !y, -x, -y,
* x + 1, y + 1, x - 1, y - 1,
* x + y, x - y, y - x,
* x & y, x | y
* on the 16-bit inputs x, y,
* according to the input bits zx, nx, zy, ny, f, no.
* In addition, computes the two output bits:
* if (out == 0) zr = 1, else zr = 0
* if (out < 0) ng = 1, else ng = 0
*/
// Implementation: Manipulates the x and y inputs
// and operates on the resulting values, as follows:
// if (zx == 1) sets x = 0 // 16-bit constant
// if (nx == 1) sets x = !x // bitwise not
// if (zy == 1) sets y = 0 // 16-bit constant
// if (ny == 1) sets y = !y // bitwise not
// if (f == 1) sets out = x + y // integer 2's complement addition
// if (f == 0) sets out = x & y // bitwise and
// if (no == 1) sets out = !out // bitwise not
CHIP ALU {
IN
x[16], y[16], // 16-bit inputs
zx, // zero the x input?
nx, // negate the x input?
zy, // zero the y input?
ny, // negate the y input?
f, // compute (out = x + y) or (out = x & y)?
no; // negate the out output?
OUT
out[16], // 16-bit output
zr, // if (out == 0) equals 1, else 0
ng; // if (out < 0) equals 1, else 0
PARTS:
Mux16(a=x, b=false, sel=zx, out=l);
Not16(in=l, out=notl);
Mux16(a=l, b=notl, sel=nx, out=m);
Mux16(a=y, b=false, sel=zy, out=n);
Not16(in=n, out=notn);
Mux16(a=n, b=notn, sel=ny, out=o);
And16(a=m, b=o, out=p);
Add16(a=m, b=o, out=q);
Mux16(a=p, b=q, sel=f, out=r);
Not16(in=r, out=notr);
Mux16(a=r, b=notr, sel=no, out[15]=s, out[0..14]=t);
Mux16(a=r, b=notr, sel=no, out[0..7]=aa, out[8..15]=bb);
Or8Way(in=aa, out=xx);
Or8Way(in=bb, out=zz);
Or(a=xx, b=zz, out=yy);
Not(in=yy, out=zr);
Mux16(a=r, b=notr, sel=no, out=out);
Mux(a=false, b=true, sel=s, out=ng);
}
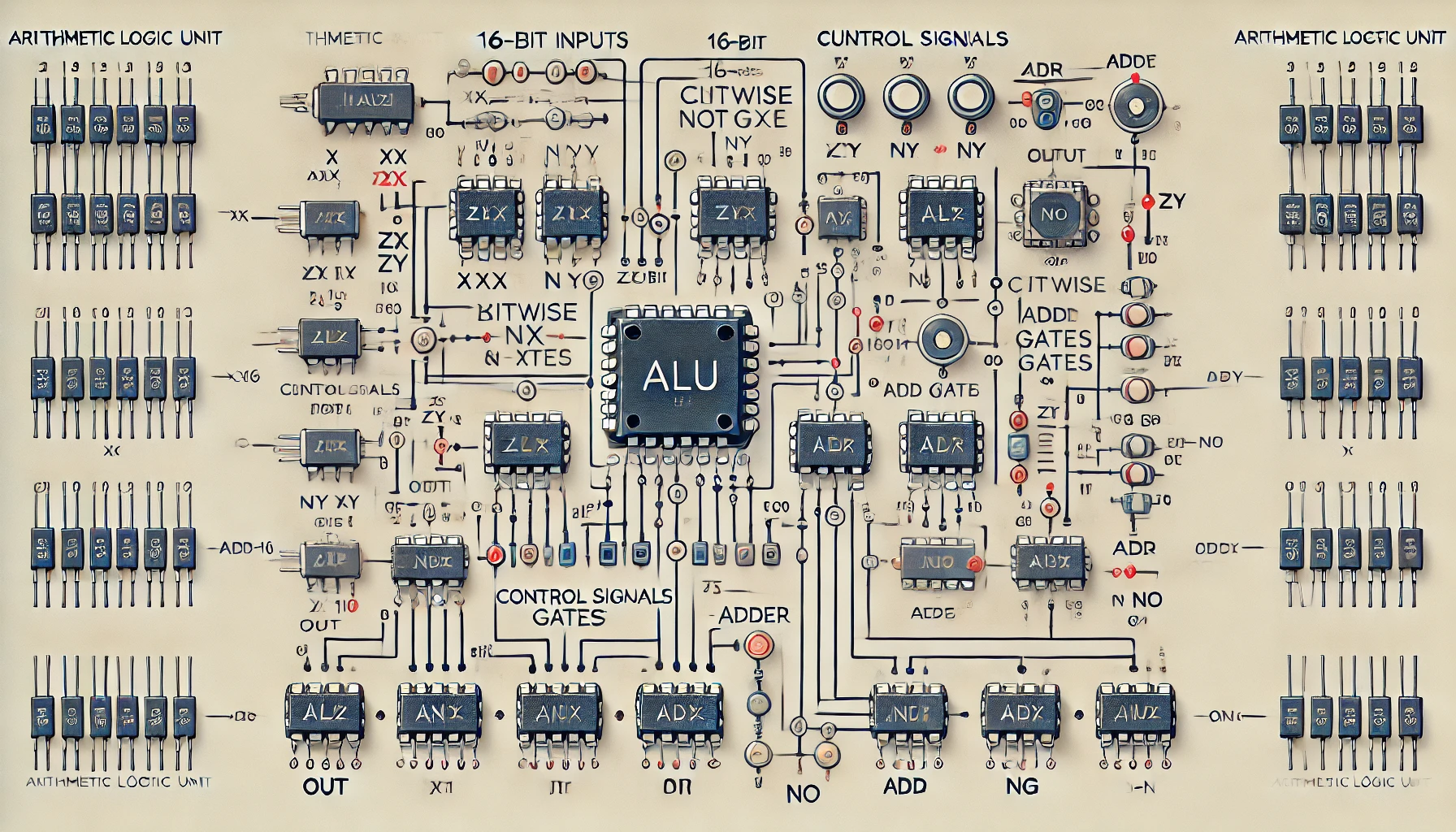
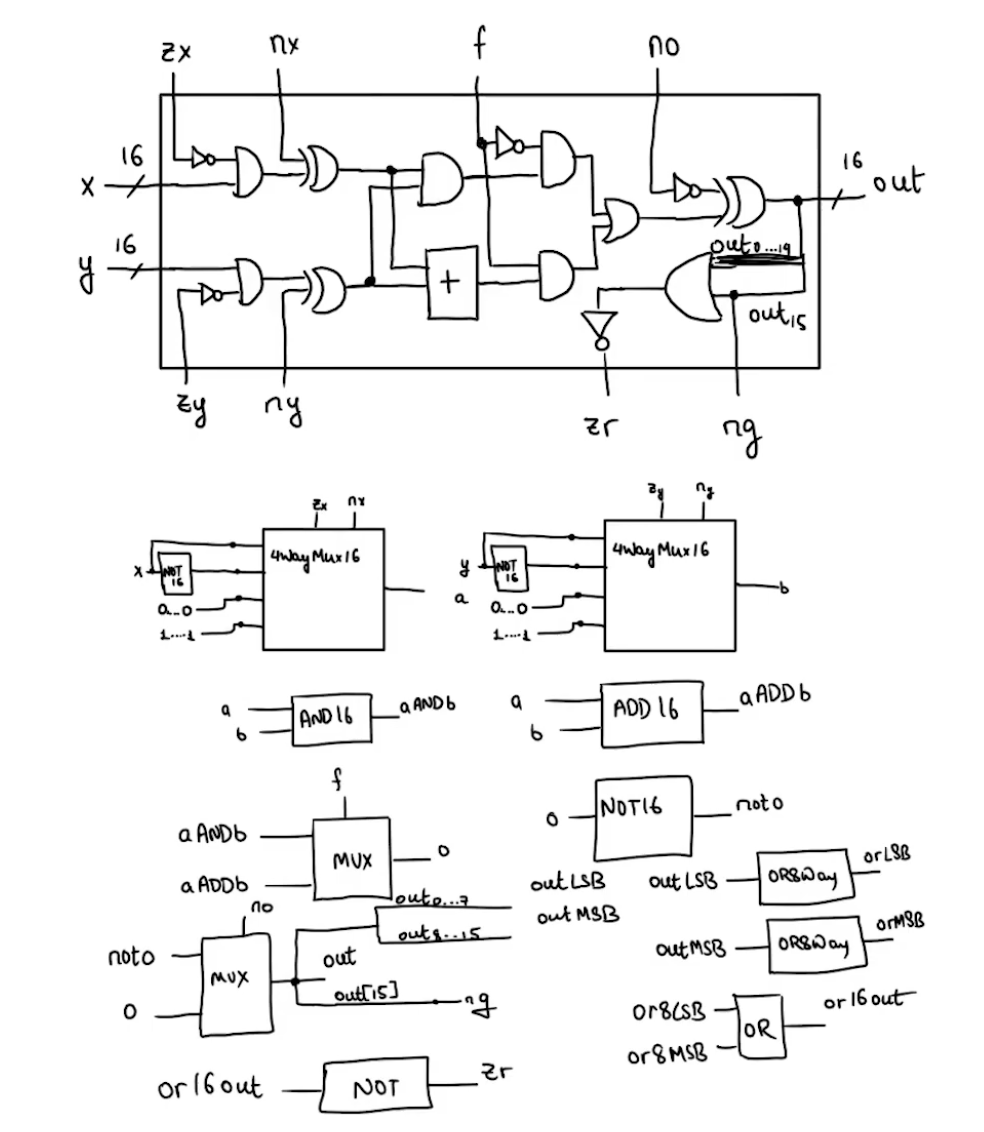
https://www.youtube.com/watch?v=Vtcm1oSSI0o