Machineboy空
Excercism - Conway's Game of Life : DFS 본문
문제요약
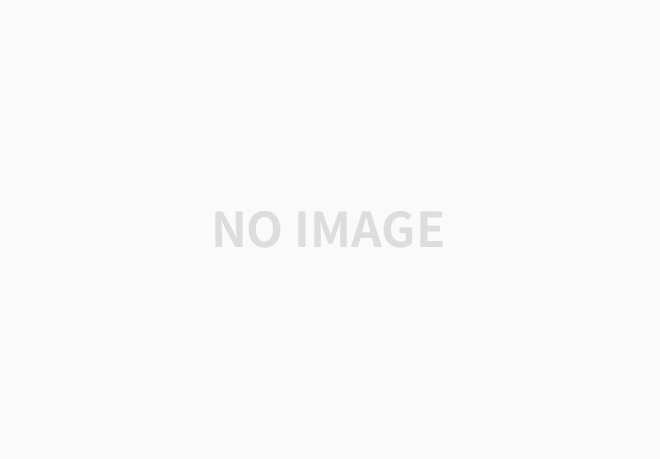
격자 8방향으로 탐색
- 현재 셀이 1 : 주변 셀 중 1이 2개 혹은 3개이면 그대로 1, 아니면 0
- 현재 셀이 0: 주변 셀 중 1이 3개이면 1, 아니면 그대로 0
https://exercism.org/tracks/csharp/exercises/game-of-life/iterations
Exercism
Learn, practice and get world-class mentoring in over 50 languages. 100% free.
exercism.org
난이도
Medium
풀이 포인트
- DFS
- 배열 복사
REVIEW
반가운 DFS 문제! DFS를 꽤 좋아하는 편이라 반가웠다.
배열이 참조타입이기 때문에 그대로 복사해서 쓸 경우 원본도 같이 훼손된다는 것을 간과해서 틀렸다..
그리고 excercism 내의 편집기로 코드를 작성하니 정렬이 어려워서 어디서 괄호가 닫히고 열리는지 파악이 되지 않아 이상한 곳에서 return을 하고 있었다..
CODE
using System;
public static class GameOfLife
{
private static readonly int[] dx = new int[] { 1, 1, 0, -1, -1, -1, 0, 1 };
private static readonly int[] dy = new int[] { 0, -1, -1, -1, 0, 1, 1, 1 };
public static int[,] Tick(int[,] matrix)
{
int rows = matrix.GetLength(0);
int cols = matrix.GetLength(1);
// 결과를 저장할 배열 생성
int[,] answer = new int[rows, cols];
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
{
int liveCellCnt = 0;
// 이웃 셀의 살아있는 개수 계산
for (int i = 0; i < 8; i++)
{
int nx = x + dx[i];
int ny = y + dy[i];
// 격자 범위를 벗어나면 무시
if (nx < 0 || ny < 0 || nx >= rows || ny >= cols) continue;
if (matrix[nx, ny] == 1) liveCellCnt++;
}
// 현재 셀이 죽은 셀인 경우
if (matrix[x, y] == 0)
{
if (liveCellCnt == 3) answer[x, y] = 1; // 죽은 셀이 부활
}
// 현재 셀이 산 셀인 경우
else
{
if (!(liveCellCnt == 2 || liveCellCnt == 3))
answer[x, y] = 0; // 산 셀이 죽음
else
answer[x, y] = 1; // 산 셀 유지
}
}
}
return answer;
}
}
'Computer > Coding Test' 카테고리의 다른 글
Excercism - Binary Search : 이진탐색, 이진탐색 트리 (0) | 2025.01.22 |
---|---|
Excercism - Resister Color : Dictionary, Array (0) | 2025.01.22 |
Excercism - Scrabble Score switch문, foreach문 (1) | 2025.01.16 |
Excercism - Roman Numerals (0) | 2025.01.15 |
Excercism - Saddle Points 2차원 배열, 최대최소 (0) | 2025.01.15 |