Machineboy空
Excercism - LargestSeriesProduct LINQ 연습 Skip Take 본문
문제요약
주어진 수를, span길이로 쪼개어 담고, 그 수의 곱 중 가장 큰 값을 반환하라.
https://exercism.org/tracks/csharp/exercises/largest-series-product/edit
Exercism
Learn, practice and get world-class mentoring in over 50 languages. 100% free.
exercism.org
난이도
Medium
풀이 포인트
- LINQ사용연습
- Any
- Select
- Max
- Skip.Take
- Aggregate
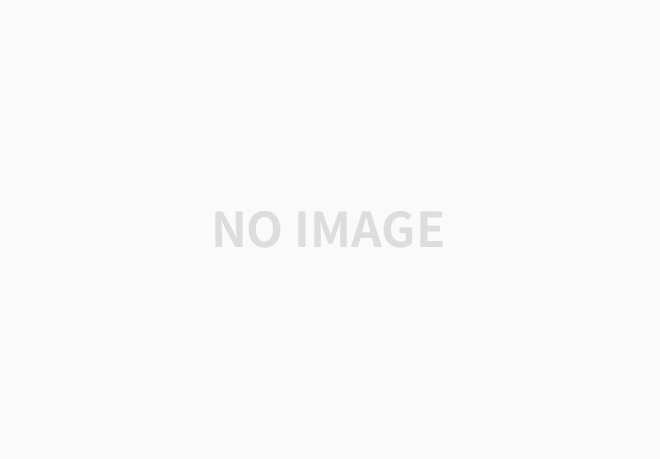
REVIEW
문제 자체는 쉬워서 Substring으로 금방 풀었으나, LINQ 연습하기 좋아보여서 기록.
LINQ는 도무지 익숙해지지가 않는다..
CODE
using System;
using System.Linq;
public static class LargestSeriesProduct
{
public static long GetLargestProduct(string digits, int span)
{
if (span > digits.Length || span < 0 || digits.Any(c => c > '9' || c < '0'))
throw new ArgumentException();
var ints = digits.Select(c => c - '0');
return Enumerable.Range(0, digits.Length - span + 1)
.Select(i => ints.Skip(i).Take(span))
.Max(s => s.Aggregate(1, (i, p) => i * p));
}
}
public static class LargestSeriesProduct
{
public static long GetLargestProduct(string digits, int span)
{
string original = digits;
List<string> list = new List<string>();
if(digits.Length < span || span < 1){
throw new ArgumentException();
}
for(int i = 0; i < digits.Length - span + 1; i++)
{
string a = original.Substring(i, span);
list.Add(a);
}
int product = -1;
foreach(string a in list)
{
int p = 1;
foreach(char c in a)
{
if(char.IsDigit(c) == false){
throw new ArgumentException();
}
int num = int.Parse(c.ToString());
p*=num;
}
product = Math.Max(product, p);
}
return product;
}
}
'Computer > Coding Test' 카테고리의 다른 글
Excercism - PhoneNumber : Regular Expressions (0) | 2025.03.04 |
---|---|
Excercism - Matrix : 2차원 배열 (0) | 2025.02.28 |
Excercism - Raindrop (0) | 2025.02.27 |
Excercism - Simple Cipher, 아스키 코드, 시저암호, 비젠네르 암호 (0) | 2025.02.26 |
Excercism - Wordy 문자열 다루기, Split, Replace, int.tryParse (0) | 2025.02.20 |