Machineboy空
C++ 문법 기초다지기 예제 모음1 - 입력, 프로세싱, 출력 본문
예제 1. 인사하기
출력 예)
What is your name? Brian
Hello, Brian, nice to meet you!
#include <iostream>
using namespace std;
string input;
int main() {
cout << "What is your name?";
cin >> input;
cout << "Hello, " + input + ", nice to meet you!";
}
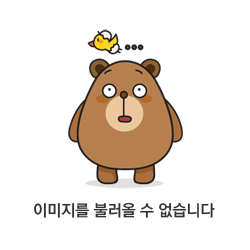
예제 2. 글자 수 세기
출력 예)
What is the input string? Homer
Homer has 5 characters.
#include <iostream>
using namespace std;
string input;
int main() {
cout << "What is the input string?";
cin >> input;
string num = to_string(input.length());
cout << input + " has " + num + " characters";
}
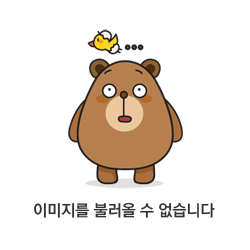
예제 3. 따옴표 출력
출력 예)
What is the quote? These aren't the droids you're looking for
Who said it? Obi-Wan Kenobi
Obi-Wan Kenobi says, "These aren't the droids you're looking for."
#include <iostream>
#include <string>
using namespace std;
// cin: 띄어쓰기 기준으로 받는다.
// 하지만 enter전까지 입력받도록 해야한다.
// cin.get(): char 하나 가져옴
// cin.getline(입력스트림객체, string 객체, 구분자) : 구분자는 입력하지 않아도 됌.
string quote, writer;
int main() {
cout << "What is the quote?\n";
getline(cin, quote);
cout << "Who said it?\n";
getline(cin, writer);
cout << writer + " says, \"" + quote + ".\"";
return 0;
}
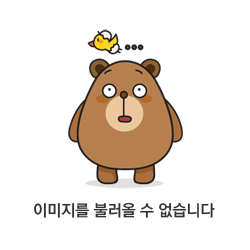
https://velog.io/@doorbals_512/C-C-%EC%9E%85%EB%A0%A5-%ED%95%A8%EC%88%98-%EC%A0%95%EB%A6%AC
[C++] C++ 입력 함수 정리
c++ 입력 함수 - cin, getline(), cin.get()
velog.io
예제 4. Mad Libs
누군가가 이야기 문장을 만드는 데 낱말이 들어갈 자리를 몇 군데 비워놓은 다음, 다른 사람이 빈 칸을 채워 전체 이야기를 만드는 것이다. 이렇게 해서 만들어진 이야기는 바보같은 내용이 되거나 웃긴 내용이 되기도 한다.
출력 예)
Enter a noun: dog
Enter a verb: walk
Enter an adjective: blue
Enter an adverb: quickly
Do you walk your blue dog quickly? That's hilarious!
#include <iostream>
#include <string>
using namespace std;
string noun, verb, adjective, adverb;
int main() {
cout << "Enter a noun: ";
cin >> noun;
cout << "Enter a verb: ";
cin >> verb;
cout << "Enter an adjective: ";
cin >> adjective;
cout << "Enter a adverb: ";
cin >> adverb;
cout << "Do you " + verb + " your " + adjective + " " + noun + " " + adjective + "? That's hilarious!";
}
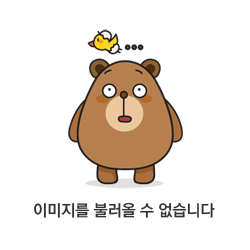
예제 5. 간단한 수학
- 제약: 문자열로 입력받아 숫자로 변환시켜 사용
출력 예)
What is the first number? 10
What is the second number? 5
10 + 5 = 15
10 - 5 = 5
10 * 5 = 50
10 / 5 = 2
#include <iostream>
#include <string>
using namespace std;
string first, second;
int main() {
cout << "What is the first number? ";
cin >> first;
cout << "What is the second number? ";
cin >> second;
int firstNum = stoi(first);
int secondNum = stoi(second);
cout << first + " + " + second + " = " + to_string(firstNum + secondNum) + "\n";
cout << first + " - " + second + " = " + to_string(firstNum - secondNum) + "\n";
cout << first + " * " + second + " = " + to_string(firstNum * secondNum) + "\n";
cout << first + " / " + second + " = " + to_string(firstNum / secondNum) + "\n";
}

예제 6. 퇴직 계산기
출력 예
What is your current age? 25
At what age would you like to retire? 65
You have 40 years left until you can retire.
It's 2015, so you can retire in 2055.
#include <iostream>
#include <string>
using namespace std;
string currentAge, retireAge;
int currentAgeNum, retireAgeNum, currentYear = 2024;
int main() {
cout << "What is your current age? ";
cin >> currentAge;
cout << "At what age would you like to retire? ";
cin >> retireAge;
currentAgeNum = stoi(currentAge);
retireAgeNum = stoi(retireAge);
cout << "You have " + to_string(retireAgeNum - currentAgeNum) + " years left until you can retire.\n";
cout << "It's " + to_string(currentYear) +", so you can retire in " + to_string(currentYear + (retireAgeNum - currentAgeNum)) + ".";
}

정리
- 입출력 관련 함수
#include <iostream>
#include <string>
using namespace std;
string input;
int main(){
cout << "Hello!";
cin >> input;
getline(cin, input);
}
- 문자열 ↔ 정수형 변환 관련
#include <iostream>
#include <string>
using namespace std;
string str;
int num;
int main() {
str = to_string(num);
num = stoi(str);
}
'Computer > Coding Test' 카테고리의 다른 글
C++ 기초 문법 다지기 예제 모음3 - 조건문 처리 (1) | 2024.10.24 |
---|---|
C++ 문법 기초다지기 예제 모음2 - 연산 (0) | 2024.10.23 |
1717: 집합의 표현 - Union-Find Tree (0) | 2024.07.22 |
15649: N과 M(1) - 백트래킹 (2) | 2024.07.22 |
입력값과 같은 구성의 수 중 가장 작은 큰 수: NextPermutation (0) | 2024.07.08 |