Machineboy空
C# Attribute, Flag Enum 본문
Attribute란?
- way to decorate a declaration to associate metadata
- to: a class, a method, an enum, a field, a property or any other supported declarations
코드의 메타데이터를 나타내는 데 사용되는 선언적 태그.
클래스, 메서드, 속성, 이벤트, 필드 등과 같은 다양한 프로그래밍 요소에 추가 정보를 제공할 때 사용
[Class]
class MyClass
{
[Field]
int myField;
}
Attribute의 역할
- only associates additional structured information
- does not modify its behavior
- to change how its target would behave or add, change or remove, restrict some its functionalities
- ex: [Flags], [Obsolete], [Conditional]
추가적인 정보를 나타낼 뿐, 보통 코드의 동작을 직접 수정하지는 않는다.
하지만 동작의 방식을 변경하거나, 추가, 제거 또는 제한하는 역할을 하기도 한다.
Flag Enum이란?
- one enum member can only refer to exactly one of those named constants
- if annotate the enum with the Flags attribute, can refer to more than one constant
enum은 보통 한 번에 하나의 상수만을 참조할 수 있지만, Flags 어트리뷰트를 붙이면 여러 개를 참조할 수 있다.
// enum은 디폴트로 int형으로 저장되기 때문에
[Flags]
enum PhoneFeatures
{
Call = 0b00000001,
Text = 0b00000010
}
// byte로 명시해줌으로써 byte로 저장되게 할 수 있음
[Flags]
enum PhoneFeatures : byte
{
Call = 0b00000001,
Text = 0b00000010
}
Flag Enum 예제
https://exercism.org/tracks/csharp/exercises/attack-of-the-trolls/edit
Exercism
Learn, practice and get world-class mentoring in over 50 languages. 100% free.
exercism.org
using System;
// TODO: define the 'AccountType' enum
enum AccountType
{
Guest,
User,
Moderator
}
// TODO: define the 'Permission' enum
[Flags]
enum Permission : byte
{
None = 0b00000000,
Read = 0b00000001,
Write = 0b00000010,
Delete = 0b00000100,
All = Permission.Read | Permission.Write | Permission.Delete
}
static class Permissions
{
public static Permission Default(AccountType accountType)
{
switch(accountType)
{
case AccountType.Guest:
return Permission.Read;
case AccountType.User:
return Permission.Read | Permission.Write;
case AccountType.Moderator:
return Permission.Read | Permission.Write | Permission.Delete;
default:
return Permission.None;
}
}
public static Permission Grant(Permission current, Permission grant)
{
return current | grant;
}
public static Permission Revoke(Permission current, Permission revoke)
{
return current & ~revoke;
}
public static bool Check(Permission current, Permission check)
{
return (current &check) == check;
}
}
https://gksid102.tistory.com/90
4. C 비트연산자(연산자 &, |, ^, ~, <<, >>, and, or, xor, 비트 반전, 비트 이동)
C 언어는 비트끼리 연산을 할 수 있는 연산자가 있습니다. 그 종류는 다음과 같습니다. 연산자 연산자의 기능 & 비트단위로 AND 연산을 한다. | 비트단위로 OR 연산을 한다. ^ 비트단위로 XOR 연산을
gksid102.tistory.com
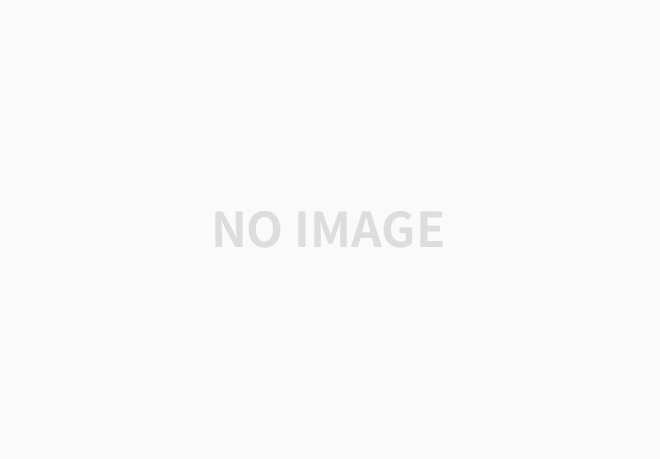
'언어 > C#' 카테고리의 다른 글
C# Type-testing operator : is, as, typeof 연산자 (0) | 2025.02.19 |
---|---|
Excercism - Yacht : LINQ 연습 (0) | 2025.02.18 |
C# 열거형 Enum (0) | 2025.02.10 |
C# IEnumerable과 lazy실행 , yield return (0) | 2025.02.07 |
C# Dictionary 딕셔너리 (0) | 2025.02.06 |